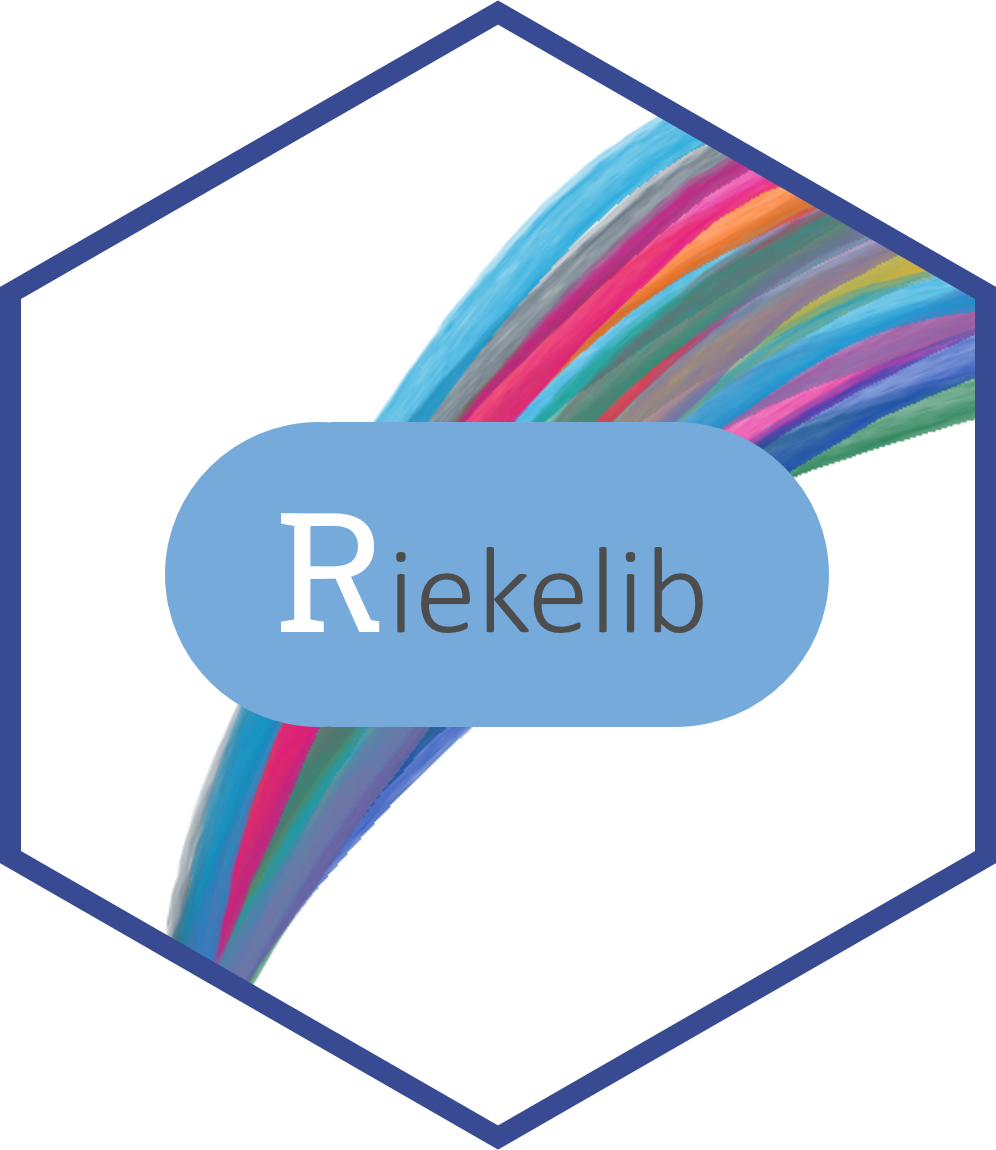
Apply a confidence interval to values in a dataframe based on the normal distribution.
Source:R/stats_extensions.R
normal_interval.Rd
Apply a confidence interval to values in a dataframe based on the normal distribution.
Arguments
- .data
Data frame; a confidence interval will be applied to each row in the data frame.
- mean
Mean of the distribution
- std_dev
Standard deviation of the distribution
- conf
Confidence interval, must be between
[0, 1]
. Defaults to 0.95.
See also
Other interval verbs:
beta_interval()
Examples
# create example df
mean <- rnorm(10, 100, 20)
std_dev <- rnorm(10, 80, 10)
example <- dplyr::bind_cols(mean, std_dev)
#> New names:
#> • `` -> `...1`
#> • `` -> `...2`
colnames(example) <- c("mean", "std_dev")
# apply the default confidence interval of 0.95
normal_interval(example, mean, std_dev)
#> # A tibble: 10 × 4
#> mean std_dev ci_lower ci_upper
#> <dbl> <dbl> <dbl> <dbl>
#> 1 70.3 75.4 -77.5 218.
#> 2 102. 93.2 -81.0 284.
#> 3 123. 79.4 -32.1 279.
#> 4 88.1 70.6 -50.3 227.
#> 5 59.1 89.9 -117. 235.
#> 6 104. 91.7 -75.5 284.
#> 7 93.7 70.2 -43.9 231.
#> 8 112. 77.9 -40.8 264.
#> 9 92.6 79.5 -63.3 248.
#> 10 91.2 77.5 -60.6 243.
# apply a custom confidence interval
normal_interval(example, mean, std_dev, conf = 0.99)
#> # A tibble: 10 × 4
#> mean std_dev ci_lower ci_upper
#> <dbl> <dbl> <dbl> <dbl>
#> 1 70.3 75.4 -124. 265.
#> 2 102. 93.2 -138. 342.
#> 3 123. 79.4 -81.0 328.
#> 4 88.1 70.6 -93.8 270.
#> 5 59.1 89.9 -172. 291.
#> 6 104. 91.7 -132. 340.
#> 7 93.7 70.2 -87.2 275.
#> 8 112. 77.9 -88.7 312.
#> 9 92.6 79.5 -112. 297.
#> 10 91.2 77.5 -108. 291.